Securely Verify Incoming Calls with BankID
Ensure the identity of the person calling you through BankID. Perfect for businesses that handle sensitive information and want to be certain of who they are talking to.
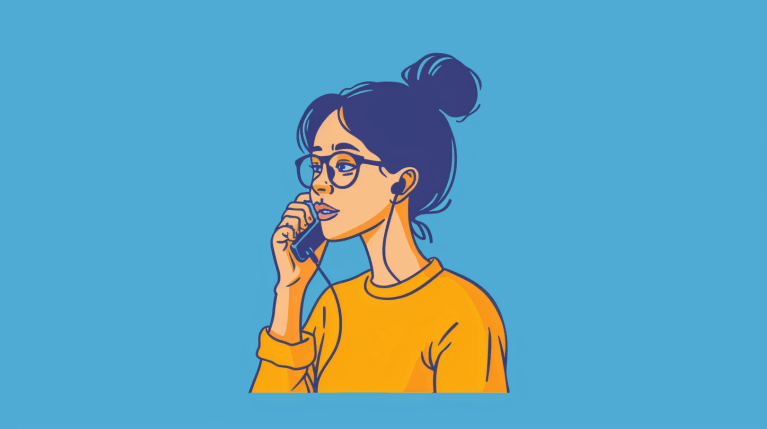
Let your customers verify themselves before speaking with someone from the team
We solve this by setting up an IVR with the 46elks API. You've probably encountered an IVR before; it's what enables 'Press 1 for customer service or press 2 for billing questions…'.
Setting up an IVR is simple and you don’t even need your own server to get started – it can be managed directly from the 46elks dashboard. However, to do something a bit more advanced like this, you need access to your own web server.
Code Example
Here's what we want to do:
- When someone calls, welcome them and explain that they need to be verified via BankID. This is done via a prerecorded audio file.
- Ask them to enter their personal number and send it to your web server to start the BankID verification.
- Once they have verified themselves, the call can be connected to your team and information about the caller can automatically be displayed.
Below is a Python code example.
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/ivr-welcome', methods=['POST'])
def ivr_welcome():
return jsonify(ivr=[
{"say": "Welcome to us. To take care of you in the best way possible, we need to verify you with BankID. Press 1 to continue."},
{"gather": {
"maxDigits": 1,
"action": "/handle-keypress"
}}
])
@app.route('/handle-keypress', methods=['POST'])
def handle_keypress():
digit_pressed = request.form['digits']
if digit_pressed == "1":
# Here you would start your BankID verification process
return jsonify(ivr=[
{"say": "Great! We're starting the verification. (This is just an example, implement the verification here.)"},
# The following line is a placeholder to connect the call after verification
{"connect": "+46123456789"}
])
else:
return jsonify(ivr=[
{"say": "Oops, it seems that you did not press 1. Please try again."},
{"redirect": "/ivr-welcome"}
])
if __name__ == '__main__':
app.run(debug=True)
This code sets up two routes: /ivr-welcome to welcome the caller and instruct them to press 1 for verification, and /handle-keypress to process their response. If they press 1, you would then proceed with the BankID verification (the details of which need to be implemented). If they press any other key, they are prompted to try again.
Note that you must implement the actual BankID integration separately and ensure that it connects properly with your IVR flow.