Make a call between a browser and a regular telephone using WebRTC
I built a thing to be able to make phone calls using WebRTC between a browser and a regular telephone. Here I will share the theoretical parts so that you will get a clearer picture on how it works.
If you don’t know what WebRTC is you can read my Swedish🇸🇪 post WebRTC for beginners.
Would you like to set up the same solution as I built? Here is the code example I wrote. If you want help getting started there are Swedish🇸🇪 step-by-step guides you can follow:
How do I make a phone call to a browser from a regular phone?
First, let’s take a step back.
Making a phone call between two browsers works the same way as sending messages between two e-mail addresses; You state the address you want to reach out to and then press “send”. This works ”out of the box” because the two addresses are on the same network (Internet).
When we make phone calls between a browser and a regular phone though, things get a bit trickier. In this case the addresses are on two completely different networks; the Internet and the telephone network. The two networks lacks knowledge about one another and are built on completely different infrastructures.
If you need an analogy, try to imagine a bus trying to swim or a boat trying to float on a rocky road - Errors will ensue.
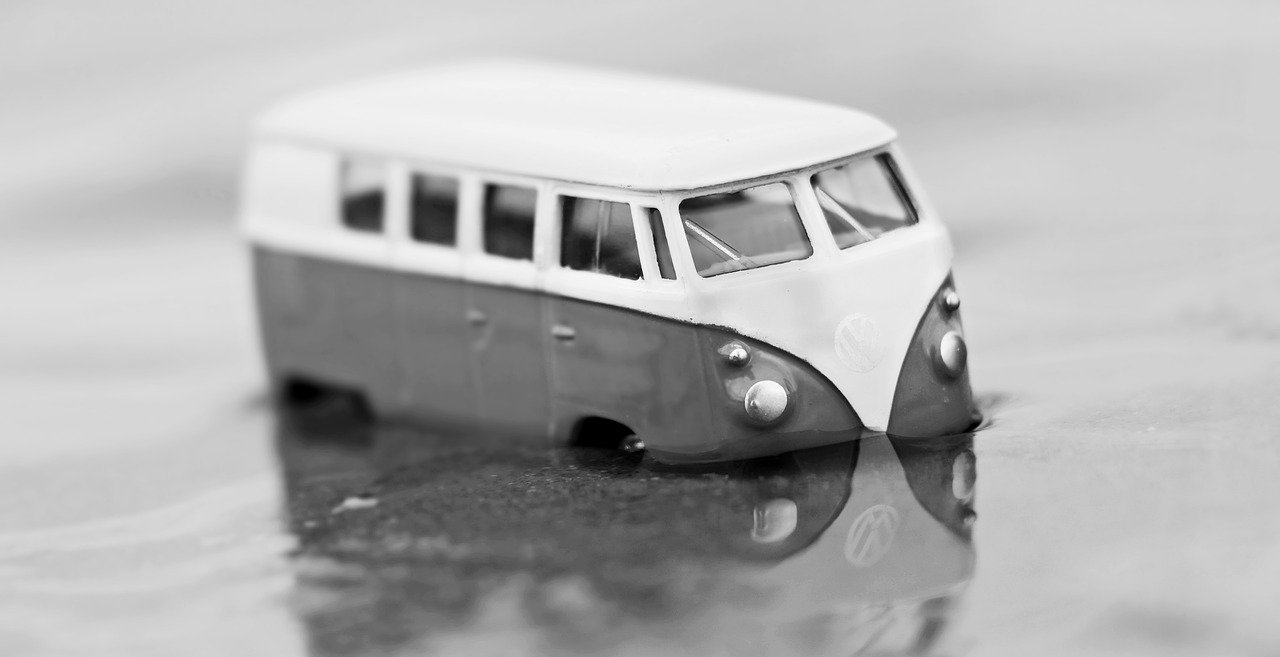
To make phone calls between these two networks we need a middleman to control the connection; Someone needs to run between the bus and the boat for them to be able to exchange information with each other. 46elks is an example of such a middleman.
So, how are you going to make a phone call to a browser? - Let’s hypothetically assume you have the following:
- A regular phone number.
The one you use on a daily basis to call/text friends and family. - A virtual phone number.
Almost like a regular phone number but with more configuration options. - A WebRTC number.
See it as a username you have on Skype, Zoom, Facetime etc.
To make a phone call to your browser you'll first make a call your virtual number. Your virtual number is pre-configured to try to contact your WebRTC number. Your browser has been instructed to listen to all communication about the WebRTC number. When your virtual number tries to contact the WebRTC number your browser will thereby react and say “incoming call”.
Let’s break this down step-by-step:
- The virtual number (+46706000001) is on the telephone network which makes it reachable for phone calls from your regular phone number.
- The fact that the number is virtual (rather than physical like your regular phone number) indicates that you can tell the number to do different things according to your needs. In this case we have told the virtual number to try to contact the WebRTC number when there are incoming calls.
- When you (or someone else) makes a phone call to your virtual number the virtual number will look at it’s config and see;
Oh! Incoming calls means I should talk to the WebRTC number
. - The virtual number then goes to the middleman and says ”Hello buddy! I have a message for the WebRTC number. Could you forward it?”.
- The middleman takes the message to the WebRTC number and says
Howdy! I have a message. Number +46706000001 is trying to reach you.
- The browser, who is told to listen to any activity about the WebRTC number, hears this message and shows a notification on the screen about an incoming call from +46706000001.
Obviously this is a simplification. There are many technical details happening behind the scenes, however we don’t have to care about those in this regard 🤓
How do I make a phone call from a browser to a regular phone?
To make a phone call from a browser is almost the same as making a phone call to a browser. The only difference is that you'll first have to call yourself to be able to call out.
Let’s assume the same premise as the previous example and suppose you have the following:
- A regular phone number.
The one you use on a daily basis to call/text friends and family. - A virtual phone number.
Almost like a regular phone number but with more configuration options. - A WebRTC number.
See it as a username you have on Skype, Zoom, Facetime etc.
Let’s break down the process step-by-step:
- To make a phone call from your browser to a phone you have to "fake” an incoming call to the browser. It can be explained like the browser seeks out a random person in town and gives that person a note which says “call me” with the phone number the browser is trying to reach, for example 123.
- The browser then asks this person to send the message to the virtual number (+46706000001).
- When the virtual number gets the message the same thing happens as in the previous example, i.e. it looks in its config and sees;
Oh! An incoming phone call means I should talk to the WebRTC number.
. - The virtual number then goes to the middleman and says ”Hello buddy! I have a message for the WebRTC number. Could you forward it?”.
- The middleman takes the message to the WebRTC number and says
Howdy! I have a message. Number +46706000001 is trying to reach you.
- The browser, who is told to listen to any activity about the WebRTC number, hears this message and reacts to the communication. In this case, however, the browser doesn’t show a notification about an “incoming call” since the browser itself triggered the phone call. Instead the browser gives a thumbs up 👍 to the middleman to indicate that it has received the phone call.
- The middleman registers the thumbs up 👍 from the browser and before it leaves it reads the message on the note and sees that it should contact another number (123). The number is called up and the conversation is put on speaker so that the browser can hear what is being said.
- Now we have successfully made a phone call from the browser to a regular phone.
In technical terms all of this means you make a so called API-call to your virtual number. The API-call could look like this in python:
import requests
auth = (
'API_USERNAME',
'API_PASSWORD'
)
fields = {
'from': '<your_virtual_number>',
'to': '<your_webrtc_number>',
'voice_start': '{"connect":"<recipient_number>"}'
}
response = requests.post(
"https://api.46elks.com/a1/calls",
data=fields,
auth=auth
)
print(response.text)
In the API-call you are sending a parameter called voice_start
(note the highlighted code above) where you enter which phone number you want to call. This is the same thing as when the browser wrote a message on a note and gave to a random person in town.
This API-call is basically the only thing that separates an outgoing phone call from an incoming phone call.
Now what?
Now it’s time for you to try out WebRTC. You do this by either build something from the scratch or start off from an existing example.
As stated in the beginning of the post I have written two Swedish🇸🇪 guides to make and receive phone calls using WebRTC with associated code examples.
Feel free to get in touch. I am curious to hear what you come up with 😁
Let's have a chat
Did you find anything especially interesting about this post, or do you have feedback to us? We are always open for discussion and eager to hear your thoughts and ideas!
Write us a comment to get in touch 👋🏼